Use the Wire Service with Base Components
To simplify record data display and manipulation and speed up development, use base components
lightning-record-*-form
components. See Compare Base Components.To create a custom user interface or if lightning-record-*-form
doesn’t meet your requirements, consider using the
wire service with other base components. For example, use the lightning-input
and lightning-formatted-*
components to build custom forms or display record
data.
Refer to the Component Reference for more information about these components.
lightning-checkbox-group
- Displays two checkboxes or more for selecting single or multiple options.
lightning-combobox
- Displays a dropdown list (picklist) of selectable options.
lightning-input
- Displays a field depending on the specified type for user input.
lightning-input-address
- Displays compound fields for address input.
- For an example of using
lightning-input
with the wire service, see theldsCreateRecord
component in the github.com/trailheadapps/lwc-recipes repo. lightning-input-location
- Displays compound fields for geolocation input.
lightning-input-name
- Displays compound fields for name input.
lightning-input-rich-text
- Displays a rich text editor with a toolbar for content formatting.
lightning-radio-group
- Displays two checkboxes or more for selecting single or multiple options.
lightning-textarea
- Displays a multiline text input field.
Although lightning-input
supports many field types,
consider other base components when you are working with different field types.
Let’s say you want to include the salutation picklist with the first and last name fields
in your user interface. You can add lightning-combobox
for the salutation picklist or use lightning-input-name
. The next example uses lightning-input-name
to display the salutation, first
name, and last name fields with their current values. To determine which base component
works best for your use case, refer to the Component
Reference.
Example: Create a Custom Form
This example creates a custom form to display and edit the values on a contact’s salutation, first name, and last name. The fields display the current values from the contact record.
lightning-record-*-form
components.<template if:true={contact.data}>
<div class="slds-m-around_medium">
<lightning-input-name
label="Contact Name"
first-name={firstname}
last-name={lastname}
salutation={salutation}
options={salutations}
class="slds-m-bottom_x-small"
required>
</lightning-input-name>
</div>
</template>
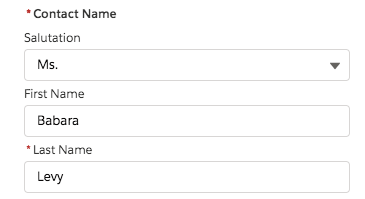
To display the initial value in the fields, use the getRecord
wire adapter.
To populate the picklist options for lightning-input-name
, use the getPicklistValues
wire adapter. This example uses the default record
type Id. To display the currently selected salutation value, import the Contact.Salutation
field reference.
import { LightningElement, api, wire } from 'lwc';
import { getRecord } from 'lightning/uiRecordApi';
import { getPicklistValues } from 'lightning/uiObjectInfoApi';
import FIRSTNAME_FIELD from '@salesforce/schema/Contact.FirstName';
import LASTNAME_FIELD from '@salesforce/schema/Contact.LastName';
import SALUTATION_FIELD from '@salesforce/schema/Contact.Salutation';
const namefields = [FIRSTNAME_FIELD, LASTNAME_FIELD, SALUTATION_FIELD];
export default class GetContactName extends LightningElement {
@api recordId; // provided by the contact record page
@wire(getPicklistValues, { recordTypeId: '012000000000000AAA', fieldApiName: SALUTATION_FIELD })
salutationValues;
@wire(getRecord, { recordId: '$recordId', fields: namefields })
contact;
get firstname() {
return this.contact.data.fields.FirstName.value;
}
get lastname() {
return this.contact.data.fields.LastName.value;
}
get salutation() {
return this.contact.data.fields.Salutation.value;
}
// creates the options array for lightning-input-name
get salutations() {
let salutationOptions = [];
Object.entries(this.salutationValues.data.values).forEach(val => {
let values = val[1];
salutationOptions.push({ 'label': values.label, 'value': values.value });
});
return salutationOptions;
}
}
Display Record Data Using Base Components
The easiest way to display record data is to use lightning-record-form
or lightning-record-view-form
. To display record data in a custom user
interface, consider using the following base components. Refer to the Component Reference for more
information on these components.
lightning-formatted-address
- For address compound fields.
lightning-formatted-address
displays an address with a link to the given location on Google Maps. The link is opened in a new tab. A static map can be displayed with the address for better context. lightning-formatted-date-time
- For date or date/time fields.
lightning-formatted-date-time
displays date and time. lightning-formatted-email
- For email fields.
lightning-formatted-email
displays an email as a hyperlink with themailto:
URL scheme. lightning-formatted-location
- For geolocation fields.
lightning-formatted-location
displays a geolocation in decimal degrees using the formatlatitude, longitude
. lightning-formatted-name
- For name fields.
lightning-formatted-name
displays a name that can include a salutation and suffix. lightning-formatted-number
- For currency, number, and percent fields.
lightning-formatted-number
displays numbers in a specified format. lightning-formatted-phone
- For phone fields.
lightning-formatted-phone
displays a phone number as a hyperlink with thetel:
URL scheme. lightning-formatted-rich-text
- For rich text fields.
lightning-formatted-rich-text
displays rich text that's formatted with allowlisted tags and attributes. lightning-formatted-text
- For text fields.
lightning-formatted-text
displays text, replaces newlines with line breaks, and adds links. lightning-formatted-time
- For time fields.
lightning-formatted-time
displays time in user's locale format. lightning-formatted-url
- For url fields.
lightning-formatted-url
displays a URL as a hyperlink.
Example: Display a Custom Record with a Map
This example displays an address on a contact record page with a static map. Clicking
the address or map opens the location on Google Maps. Since the lightning-record-form
and lightning-record-view-form
components don’t provide a static map, the
example uses the lightning-formatted-address
component.
<template>
<lightning-card
title="Display Contact Address"
icon-name="standard:contact">
<template if:true={contact.data}>
<div class="slds-m-around_medium">
<lightning-formatted-address
street={street}
city={city}
country={country}
province={state}
postal-code={postal}
show-static-map
></lightning-formatted-address>
</div>
</template>
</lightning-card>
</template>
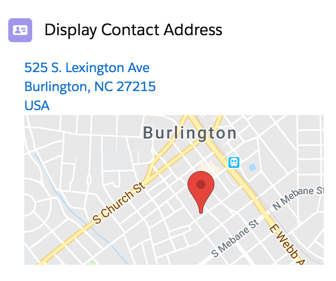
To display address compound fields, use the getRecord
wire adapter.
import { LightningElement, api, wire } from 'lwc';
import { getRecord } from 'lightning/uiRecordApi';
import STREET_FIELD from '@salesforce/schema/Contact.MailingStreet';
import CITY_FIELD from '@salesforce/schema/Contact.MailingCity';
import STATE_FIELD from '@salesforce/schema/Contact.MailingState';
import COUNTRY_FIELD from '@salesforce/schema/Contact.MailingCountry';
import POSTAL_FIELD from '@salesforce/schema/Contact.MailingPostalCode';
const FIELDS = [STREET_FIELD, CITY_FIELD, STATE_FIELD, COUNTRY_FIELD, POSTAL_FIELD];
export default class GetContactAddress extends LightningElement {
@api recordId; // provided by the contact record page
@wire(getRecord, { recordId: '$recordId', fields: FIELDS })
contact;
get street() {
return this.contact.data.fields.MailingStreet.value;
}
get city() {
return this.contact.data.fields.MailingCity.value;
}
get state() {
return this.contact.data.fields.MailingState.value;
}
get country() {
return this.contact.data.fields.MailingCountry.value;
}
get postal() {
return this.contact.data.fields.MailingPostalCode.value;
}
}
No comments:
Post a Comment